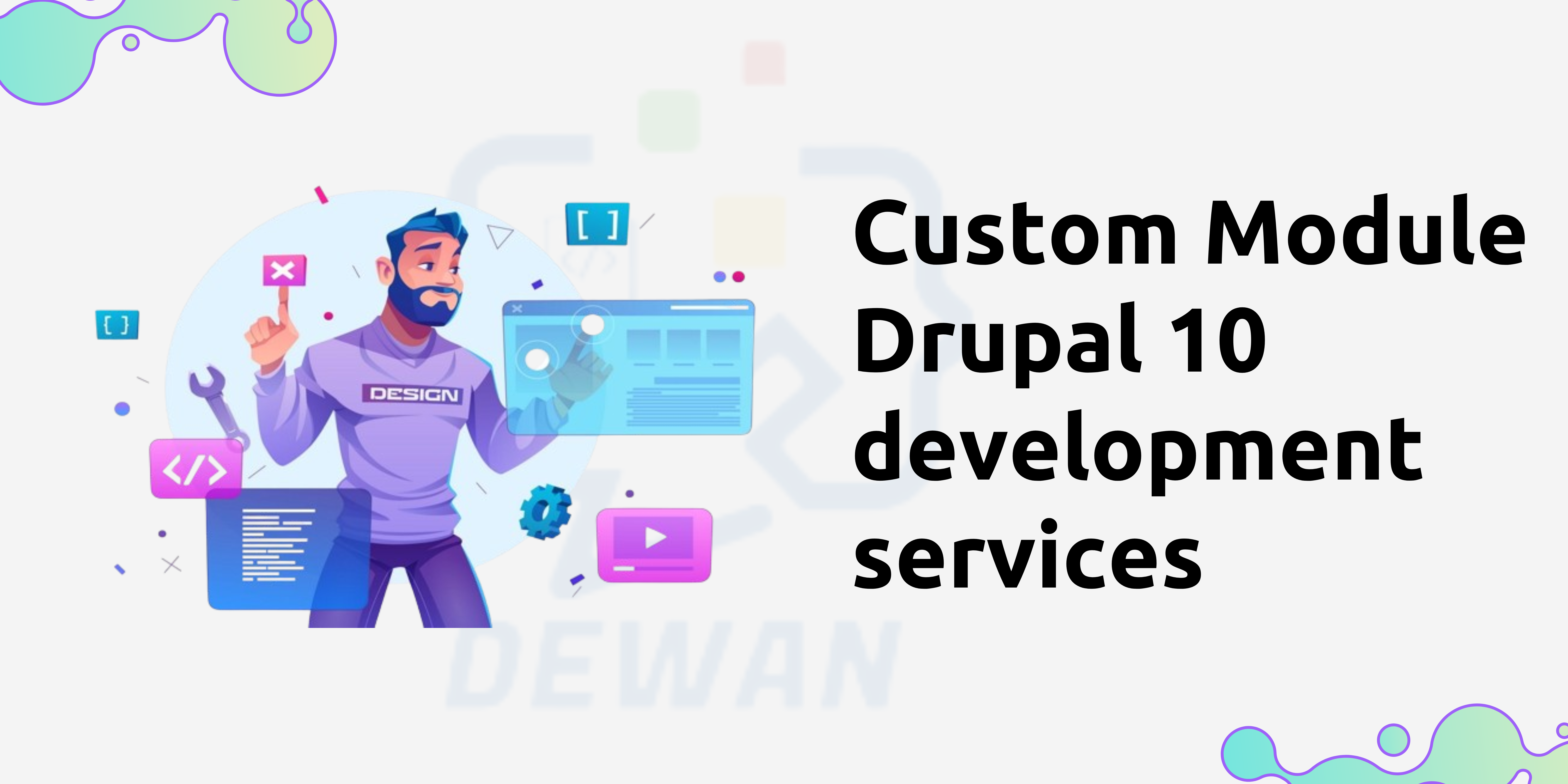
Introduction
Create a custom module in Drupal 10 lets in you to extend and customize the functionality of your Drupal site. In this advent, permit's cowl the fundamental steps and issues for constructing a fundamental custom module.
Set Up the Module organization
Create Module Folder
IInside the modules/custom listing of your Drupal set up, create a brand new folder on your module. Select a significant and particular call on your module, ideally in lowercase with underscores (e.g., custom module).
Create the Module information Document
Create .info.yml File
Inner your module folder, create a record named custom_module.data.yml. This report consists of metadata about your module.
Create the Module PHP File
Create .module File
Inside the equal module folder, create a file named custom_module.module. This document will incorporate the personal home page code to your module.
Create module_name.links.menu.yml
The module_name.links.menu.yml file in a Drupal module is used to define menu links as a way to seem in Drupal's navigation menus. Here's an example of how you can create a module_name.links.menu.yml file.
In this example:
module_name.example_rout
This is the device name of your menu hyperlink. It should be precise within the scope of your module.
Title
The textual content with a purpose to be displayed as the menu hyperlink.
Description
A brief description of the menu link.
Parent
The device name of the parent menu link. In this situation, it is set to foremost-menu to make it a top-level menu link. in case you want it to be a submenu, you can specify the gadget call of the parent menu link.
route_name
The Drupal path name associated with the menu hyperlink. It corresponds to the direction defined for your module. In the instance, it's set to module_name.example_route.
menu_name
The name of the menu in which the hyperlink may be positioned. In this example, it is set to important-menu.
You may also like to read : Salesforce Integration With Drupal
Weight
The burden of the menu hyperlink, influencing its role among different links. A decrease weight value way it'll seem better within the menu. Don't forget to replace module_name.example_route with the real direction call you have defined to your module. This YAML document gives Drupal with the vital facts to show your custom menu hyperlink within the specific menu. Regulate the parameters in keeping with your module's necessities.
Implement Essential Hooks
Implement hook_help()
Internal your custom_module.module file, enforce a simple hook_help() to provide information approximately your module.
Enable the Module
Find and Enable
Find your module in the listing, take a look at the checkbox next to it, and click the "install" button to allow the module.
Verify Your Module
Congratulations! you've got simply created a fundamental custom module in Drupal 10. This is a place to begin, and you can make bigger your module's functionality with the aid of implementing additional hooks, developing templates, or adding custom capabilities primarily based to your requirements.
Drupal Custom Module Assurance and Compatibility
Module Assurance in Drupal
While growing Drupal modules, it's commonplace to rely on the functionality provided via other modules. Module dependencies assist you to declare these dependencies explicitly. Right here are greater details on handling module dependencies.
Declare Dependencies
Within the .info.yml report of your module, use the dependencies key to list the modules your module relies upon on.
For example
Dependency Types
Drupal modules can rely upon different modules or specific sub-modules of a module. The format is module_name:submodule for sub-modules.
Checking Dependencies
While you enable your module, Drupal assessments if the specified dependencies are met. If any dependencies are lacking or not met, Drupal prevents your module from being enabled.
Recursive Dependencies
Drupal robotically includes dependencies of enabled modules, making it pointless to allow every dependency manually.
Module Unity in Drupal 10
Ensuring that your custom module is well matched with the version of Drupal center you are the usage of is important. Right here are extra insights into dealing with module compatibility.
core_version_requirement
The core_version_requirement key within the .info.yml record specifies the variety of Drupal middle versions your module is compatible with.
Semantic Versioning
Drupal follows semantic versioning. The ^ image indicates compatibility with any Drupal core model that includes the required essential model.
Upgrad The Custom Modules
Frequently update your custom modules to make certain compatibility with the state-of-the-art Drupal middle releases. Drupal's core APIs might also evolve, and your modules have to adapt as a consequence.
Compatibility Testing
Check your modules on special Drupal center variations throughout improvement and earlier than deploying modifications to manufacturing. This enables perceive and remedy compatibility problems early.
Documentation
Virtually file module dependencies inside the README or module documentation.
Dependency Injection
Utilize Drupal's dependency injection machine whilst interacting with external modules or offerings.
Community Collaboration
Interact with the Drupal community forums and documentation to live informed approximately first-rate practices and community-contributed modules. information and managing dependencies and compatibility is critical for retaining a stable and scalable Drupal site. regularly test for updates, comply with Drupal's coding requirements, and contribute returned to the network whilst feasible.
Use Item Orientated Programing (OOP)
Whilst developing custom modules in Drupal, embracing object-orientated Programming (OOP) standards can lead to greater maintainable and modular code. let's discover the way to incorporate OOP right into a Drupal custom module.
Item-Oriented Programming in Drupal Custom Modules
Drupal, being constructed on PHP, supports object-oriented programming. Incorporating OOP standards into your custom module can enhance code enterprise, reusability, and maintainability.
Step 1: Create a Class for Your Module
File Structure
Internal your custom module folder, create a src folder. inside this folder, create a PHP file to your elegance. For instance, CustomModule.Hypertext Preprocessor.
Namespace and Class Declaration
In your PHP file, define a namespace and declare a class for your module.
<?php
namespace Drupal\custom_module;
/**
* Defines the CustomModule class.
*/
Class custommodule {
/**
* Constructor for CustomModule.
*/
public function __construct() {
// Constructor logic, if needed.
}
/**
* Example method.
*/
public function exampleMethod() {
return 'Hello, this is an example method.';
}
}
Utilize Dependency Injection
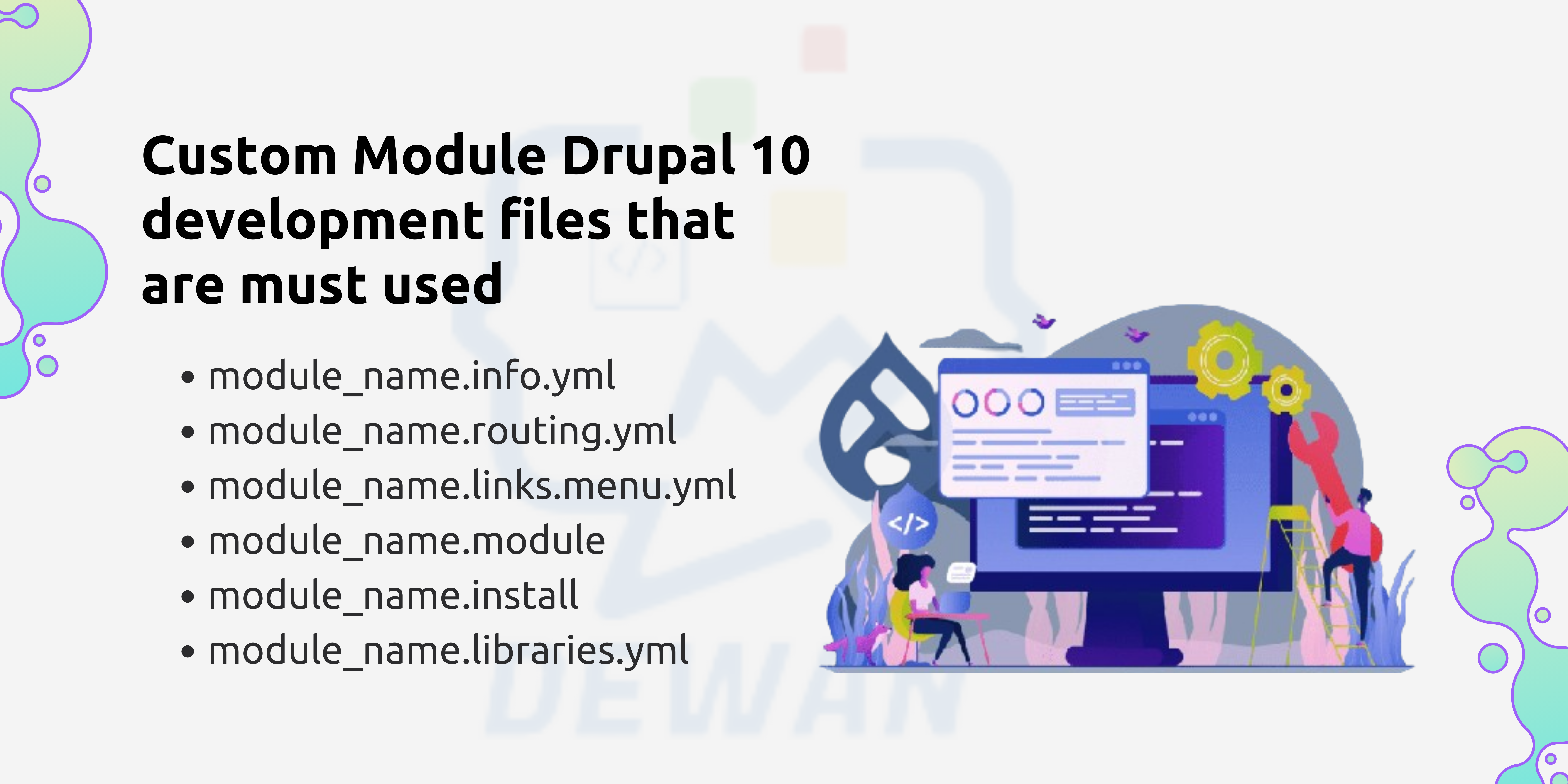
Constructor Parameters
Leverage dependency injection through passing vital services or dependencies as constructor parameters.
namespace Drupal\custom_module;
use Drupal\Core\Config\ConfigFactoryInterface;
/**
* Defines the CustomModule class.
*/
class CustomModule {
/**
* Configuration service.
*
* @var \Drupal\Core\Config\ConfigFactoryInterface
*/
protected $configFactory;
/**
* Constructor for CustomModule.
*
* @param \Drupal\Core\Config\ConfigFactoryInterface $config_factory
* The configuration factory service.
*/
public function __construct(ConfigFactoryInterface $config_factory) {
$this->configFactory = $config_factory;
}
// ... rest of the class
}
Integrate OOP with Drupal Hooks
Example Hook Implementation
Integrate OOP principles when implementing Drupal hooks within your class.
<?php
namespace Drupal\custom_module;
use Drupal\Core\Config\ConfigFactoryInterface;
/**
* Defines the CustomModule class.
*/
class CustomModule {
// ... constructor and other methods
/**
* Implements hook_help().
*/
public function help() {
return [
'#markup' => '<p>' . t('A custom Drupal module.') . '</p>',
];
}
}
Instantiate Your Class
Module File
In your custom_module.module file, instantiate your class and call methods as needed.
<?php
use Drupal\Core\Routing\RouteMatchInterface;
/**
* Implements hook_help().
*/
function custom_module_help($route_name, RouteMatchInterface $route_match) {
// Instantiate the CustomModule class.
$customModule = new \Drupal\custom_module\CustomModule();
// Call methods from the instantiated class.
$helpContent = $customModule->help();
return $helpContent;
}
Truly! while growing custom modules in Drupal, embracing item-orientated Programming (OOP) principles can lead to extra maintainable and modular code. permit's discover a way to comprise OOP into a Drupal custom module.
Object-Oriented Programming in Drupal Custom Modules
Drupal, being constructed on PHP, helps object-oriented programming. Incorporating OOP ideas into your custom module can enhance code company, reusability, and maintainability.
Step 1: Create a Class for Your Module
File Structure
Inside your custom module folder, create a src folder. Inside this folder, create a PHP document to your class. As an instance, CustomModule.php.
1.2 Namespace and Class Declaration
In your PHP file, define a namespace and declare a class for your module:
php
<?php
namespace Drupal\custom_module;
/**
* Defines the CustomModule class.
*/
class CustomModule {
/**
* Constructor for CustomModule.
*/
public function __construct() {
// Constructor logic, if needed.
}
/**
* Example method.
*/
public function exampleMethod() {
return 'Hello, this is an example method.’
}
}
Step 2: Utilize Dependency Injection
Constructor Parameters
Leverage dependency injection by passing necessary services or dependencies as constructor parameters. php.
<?php
namespace Drupal\custom_module;
use Drupal\Core\Config\ConfigFactoryInterface;
/**
* Defines the CustomModule class.
*/
class CustomModule {
/**
* Configuration service.
*
* @var \Drupal\Core\Config\ConfigFactoryInterface
*/
protected $configFactory;
/**
* Constructor for CustomModule.
*
* @param \Drupal\Core\Config\ConfigFactoryInterface $config_factory
* The configuration factory service.
*/
public function __construct(ConfigFactoryInterface $config_factory) {
$this->configFactory = $config_factory;
}
// ... rest of the class
}
Step 3: Integrate OOP with Drupal Hooks
3.1 Example Hook Implementation
Integrate OOP principles when implementing Drupal hooks within your class.
<?php
namespace Drupal\custom_module;
use Drupal\Core\Config\ConfigFactoryInterface;
/**
* Defines the CustomModule class.
*/
class CustomModule {
// ... constructor and other methods
/**
* Implements hook_help().
*/
public function help() {
return [
'#markup' => '<p>' . t('A custom Drupal module.') . '</p>',
];
}
}
Step 4: Instantiate Your Class
4.1 Module File:
In your custom_module.module file, instantiate your class and call methods as needed:
php
<?php
use Drupal\Core\Routing\RouteMatchInterface;
/**
* Implements hook_help().
*/
function custom_module_help($route_name, RouteMatchInterface $route_match) {
// Instantiate the CustomModule class.
$customModule = new \Drupal\custom_module\CustomModule();
// Call methods from the instantiated class.
$helpContent = $customModule->help();
return $helpContent;
}
Advantages of OOP in Drupal custom Modules
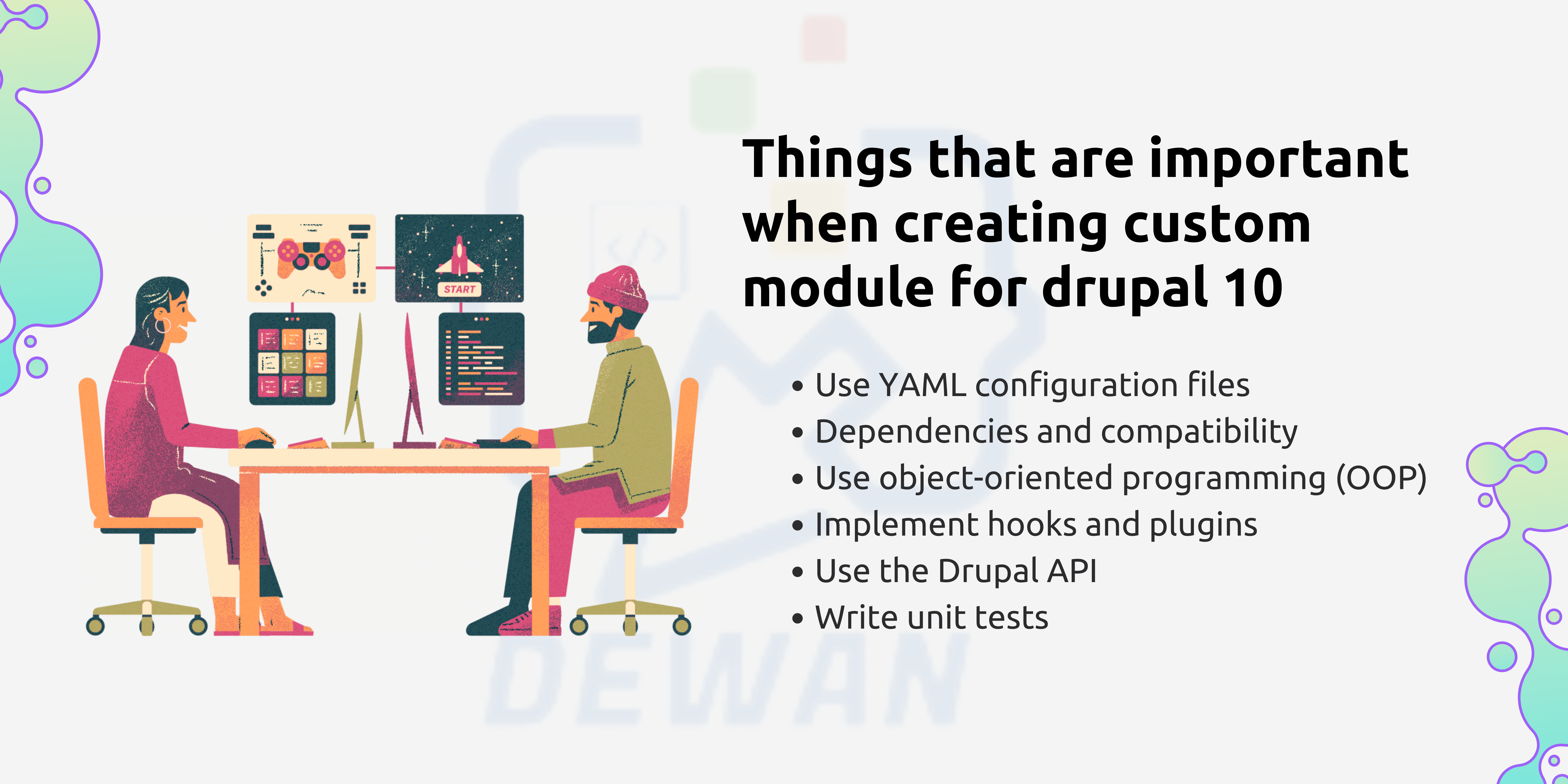
Code Management
OOP promotes a based method, making it less difficult to organize and manage code.
Reusability
Well-designed training can be reused in specific components of your module or even in different tasks.
Dependency Control
Dependency injection helps control dependencies and makes trying out less difficult.
Extendibility
OOP allows for easy extension of capability via growing new instructions or obtaining from current ones.
Conclusion
Managing with module dependencies and making sure compatibility are important factors of developing strong and maintainable Drupal packages. by using following pleasant practices and information the Drupal architecture, you can create custom modules that seamlessly combine with the Drupal environment.